Curve Control Creator¶
The control module provides functions and a graphical interface to create, manipulate, import and export curve controls.
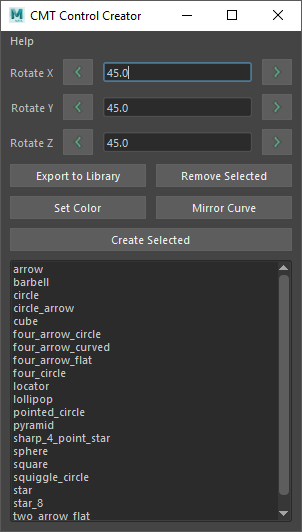
The APIs provided allow curve shapes to be abstracted from transforms. This allows the creation of rigging constructs independent of actual curve shapes which can vary greatly from asset to asset. The general workflow would be to create rig controls with transforms only without shapes. After the rigs are created, add shapes to the transforms with this tool/API. The shapes can then be serialized to disk to load back in an automated build.
Example Usage¶
The Control Creator tool can be accessed in the cmt menu:
CMT > Rigging > Control Creator
API¶
import cmt.rig.control as control
curve = cmds.circle()[0]
# Save the curve to disk
file_path = "{}/control.json".format(cmds.workspace(q=True, rd=True))
control.export_curves([curve], file_path)
# Load the curve back in
cmds.file(n=True, f=True)
control.import_curves(file_path)
# Create another copy of the curve
control.import_new_curves(file_path)
# Create the curve on the selected transform
node = cmds.createNode('transform', name='newNode')
control.import_curves_on_selected(file_path)
# Manipulate the curve before creating
curve = control.load_curves(file_path)[0]
curve.scale_by(2, 2, 2)
curve.set_rotation(0, 60, 0)
curve.set_translation(10, 5, 0)
new_node = curve.create("anotherNode")
# Mirror the curve
mirrored = cmds.createNode("transform", name="mirroredNode")
cmds.setAttr("{}.t".format(mirrored), -10, -5, 2)
cmds.setAttr("{}.r".format(mirrored), -55, 10, 63)
control.mirror_curve(new_node, mirrored)
-
class
cmt.rig.control.
CurveShape
(transform=None, cvs=None, degree=3, form=0, knots=None, color=None)[source]¶ Represents the data required to build a nurbs curve shape
-
create
(transform=None, as_controller=True)[source]¶ Create a curve.
- Parameters
transform – Name of the transform to create the curve shape under. If the transform does not exist, it will be created.
as_controller – True to mark the curve transform as a controller.
- Returns
The transform of the new curve shapes.
-
rotate_by
(x, y, z, local=True)[source]¶ Rotate the curve cvs by the given euler rotation values
- Parameters
x – Rotate X
y – Rotate Y
z – Rotate Z
local – True for local space, False for world
-
scale_by
(x, y, z, local=True)[source]¶ Scale the curve cvs by the given amount.
- Parameters
x – Scale X
y – Scale Y
z – Scale Z
local – True for local space, False for world
-
set_rotation
(x, y, z)[source]¶ Set the absolute rotation of the curve shape in euler rotations.
- Parameters
x – Rotate X
y – Rotate Y
z – Rotate Z
-
set_scale
(x, y, z, local=True)[source]¶ Set the absolute scale of the curve shape.
- Parameters
x – Scale X
y – Scale Y
z – Scale Z
local – True for local space, False for world
-
-
cmt.rig.control.
export_curves
(controls=None, file_path=None)[source]¶ Serializes the given curves into the control library.
- Parameters
controls – Optional list of controls to export. If no controls are specified, the selected curves will be exported.
file_path – File path to export to
- Returns
The exported list of ControlShapes.
-
cmt.rig.control.
import_curves_on_selected
(file_path=None, tag_as_controller=False)[source]¶ Imports a control shape from disk onto the selected transform.
- Parameters
file_path – Path to the control file.
tag_as_controller – True to tag the curve transform as a controller
- Returns
The new curve transform
-
cmt.rig.control.
import_new_curves
(file_path=None, tag_as_controller=False)[source]¶ Imports control shapes from disk onto new transforms.
- Parameters
file_path – Path to the control file.
tag_as_controller – True to tag the curve transform as a controller
- Returns
The new curve transforms
-
cmt.rig.control.
load_curves
(file_path=None)[source]¶ Load the CurveShape objects from disk.
- Parameters
file_path –
- Returns
-
cmt.rig.control.
mirror_curve
(source, destination)[source]¶ Mirrors the curve on source across the YZ plane to destination.
The cvs will be mirrored in world space no matter the transform of destination.
- Parameters
source – Source transform
destination – Destination transform
- Returns
The mirrored CurveShape object